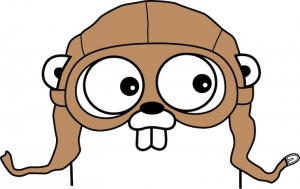
Go is a promising language. It's a strong replacement for Java, it's similarly productive to writing Python, and it is an excellent tool for writing servers.
I'm starting to dive into it in my spare time, and in this blog post I will show you the basics of getting started with Go and Redis together. This tutorial assumes you're running a flavor of Mac OS X and are comfortable with Terminal.
Run the following commands on most *nix command lines to download, unpack and install Redis:
wget http://download.redis.io/redis-stable.tar.gz
tar xvzf redis-stable.tar.gz
cd redis-stable
make
sudo make install
Install Go. As of writing,
this was the latest version for Mac.
(You can find the list of all available downloads by clicking from
the go download page.)
You must have Mercurial installed for the
go get
command to work. So let's install Mercurial, which is super easy if you manage packages with
Homebrew.
brew update
brew doctor
brew install mercurial
Create your go workspace. This is just the way go works. Do the following:
mkdir gocode
Open up .bashrc (or .zshrc if using zsh):
vim .bashrc
On the last line set the GOPATH:
export GOPATH="$HOME/gocode"
Now, we can create our project workspace.
cd gocode
mkdir -p src/github.com/yourusername
Cool, now let's create the redis go project.
cd src/github/yourusername
mkdir hello-go-redis
cd hello-go-redis
vim hello-go-redis.go
Paste the following into
hello-go-redis.go.
package main
import "fmt"
import "github.com/garyburd/redigo/redis"
func main() {
//INIT OMIT
c, err := redis.Dial("tcp", ":6379")
if err != nil {
panic(err)
}
defer c.Close()
//set
c.Do("SET", "message1", "Hello World")
//get
world, err := redis.String(c.Do("GET", "message1"))
if err != nil {
fmt.Println("key not found")
}
fmt.Println(world)
//ENDINIT OMIT
}
go get github.com/garyburd/redigo/redis
/usr/local/bin/redis-server
go run hello-go-redis.go
You will get the message back "Hello World." Nice job, you just wrote your first Go script. Check out further tutorials on the
Go site.