Twilio SendGrid
Everything you need to deliver emails at scale
-
148+ billion emails sent every month
-
82,000+ customers
-
100+ full-time email deliverability experts
No credit card required | Get started quickly | Access to all Twilio products
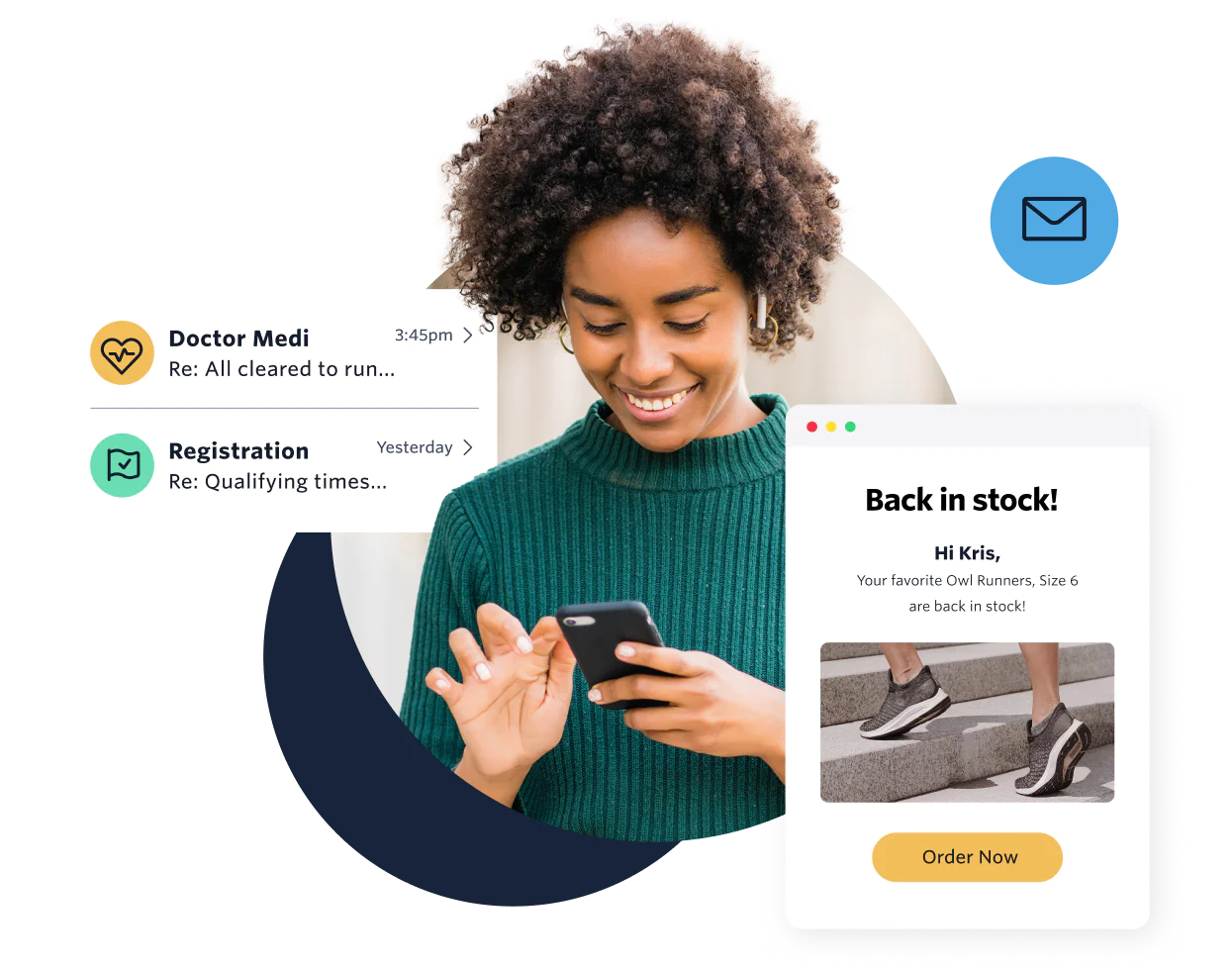
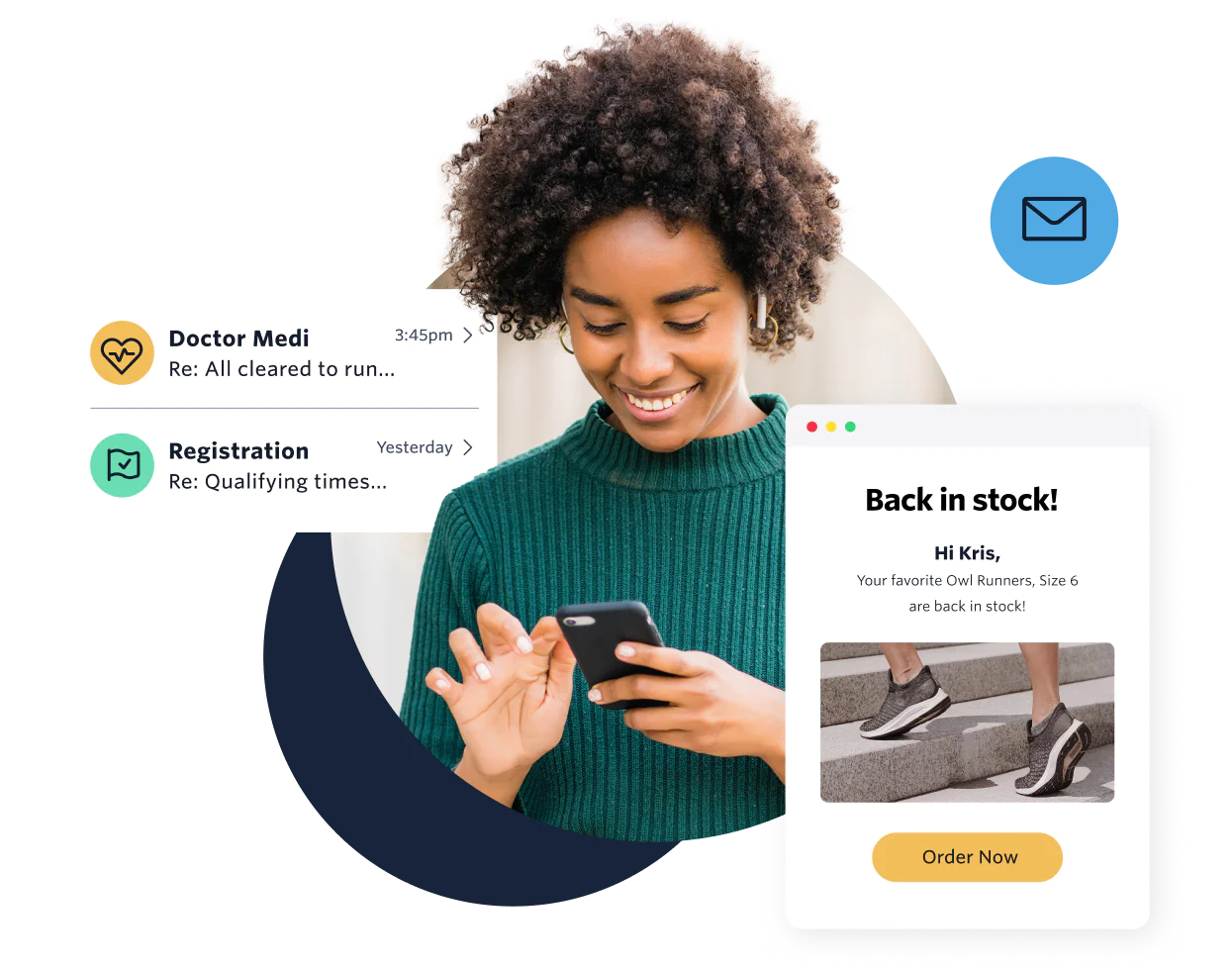